Optimizing performance in NEXT.js: Tips and tricks
Davis Gitonga / January 19, 2023
15 min read •
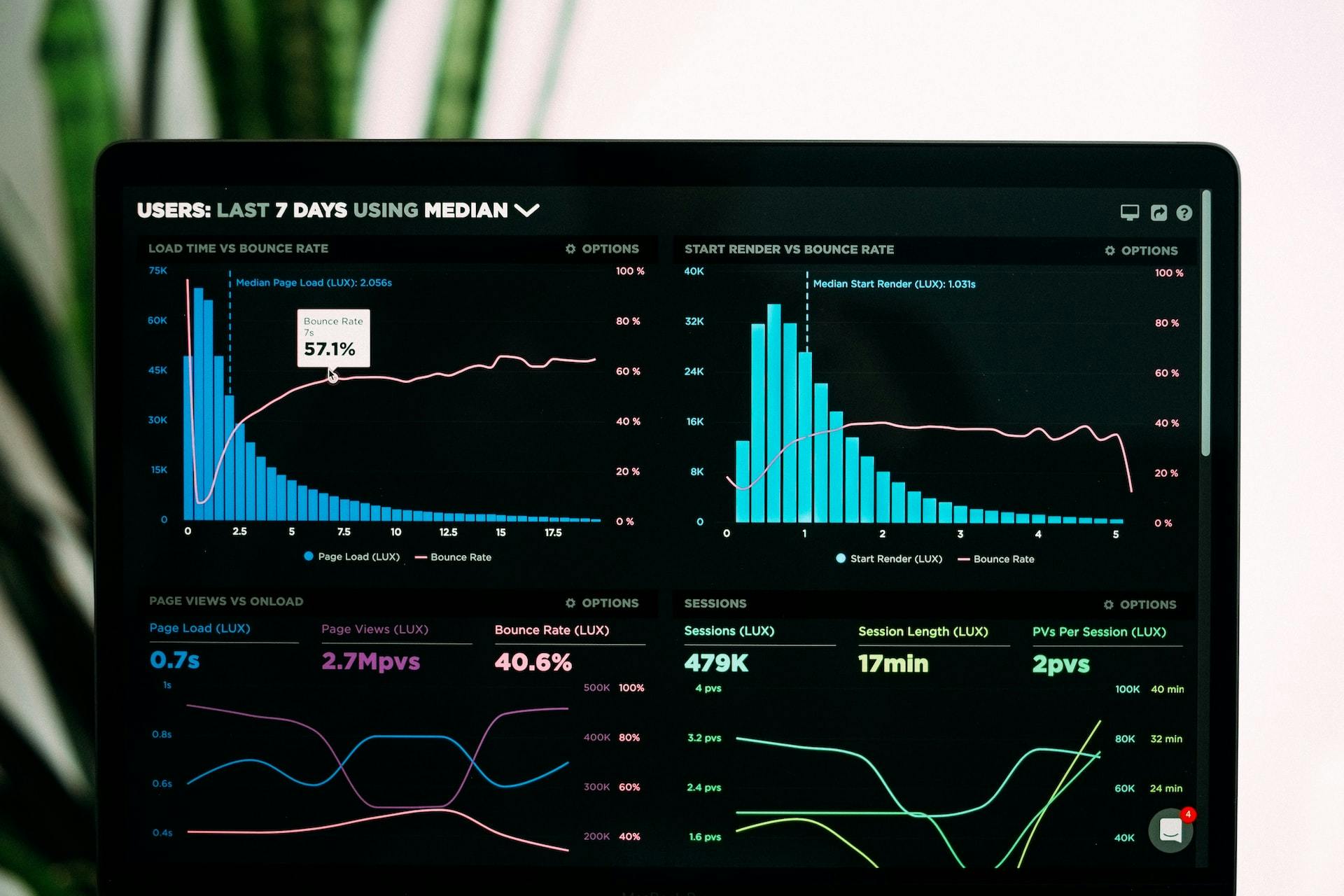
Photo by Luke Chesser
Introduction
Performance optimization is an essential aspect of web development, and this is especially true when it comes to building applications with NEXT.js. As a server-rendered React framework, NEXT.js has the ability to deliver fast and efficient apps, but this requires some careful consideration and attention to detail.
In this blog, we'll be exploring a range of tips and tricks for optimizing the performance of your NEXT.js app. From automatic code splitting and client-side rendering to image optimization and caching, we'll cover everything you need to know to get the most out of your NEXT.js app.
So if you're ready to take your NEXT.js app to the next level (pun intended), grab a coffee, and let's get started! Just try not to get too excited about all the performance gains you're about to achieve – we don't want you to spill that coffee all over your keyboard. Trust us, we've been there.
1. Use automatic code splitting
One of the most important ways to improve the performance of your NEXT.js app is to use automatic code splitting. But what exactly is code splitting, and how does it work in NEXT.js?
The process of breaking up your app's code into smaller, more manageable chunks that can be loaded on demand rather than all at once is known as code splitting. This offers a number of advantages, including smaller bundle sizes, faster initial load times, and more efficient bandwidth utilization.
Code splitting in NEXT.js is accomplished through the use of dynamic
import syntax, which allows you to import a module only when it is required. Assume you have a large library that is only used in a few pages of your app. Instead of including the entire library in the main bundle, dynamic
imports can be used to load the library only when those specific pages are visited.
To use dynamic
imports in your NEXT.js app, simply wrap the import statement in a function and call that function when you need to load the module. For example:
import dynamic from 'next/dynamic';
const MyComponent = dynamic(() => import('./my-component'));
In addition to dynamic
imports, NEXT.js includes the next/dynamic
component, which allows you to easily create dynamically loaded components. This is especially useful for lazy-loading large or complex components that do not require immediate loading. For example:
import dynamic from 'next/dynamic'
const MyComponent = dynamic(
() => import('./my-component'),
{ loading: () => <p>Loading...</p> }
)
Overall, code splitting is an important tool for optimizing the performance of your NEXT.js app, and the dynamic
import syntax and next/dynamic
component make it easy to implement in your projects. So give it a try and see the performance benefits for yourself!
2. Enable client-side rendering
Enabling client-side rendering, also known as partial hydration, is another way to boost the performance of your NEXT.js app. This is the process of rendering a server-rendered app on the client side, allowing specific parts of the app to be updated interactively without having to reload the entire page.
Client-side rendering in NEXT.js is accomplished through the use of the getInitialProps method, which is a lifecycle method that is invoked on both the server and the client. getInitialProps
enables server-side rendering in a page and allows you to do initial data population, which means sending the page with the data from the server already populated. This is particularly useful for SEO.
getInitialProps
will disable Automatic Static Optimization.
Suppose you have a component that displays a list of recent blog posts. To enable client-side rendering for this component, use the getInitialProps
method to retrieve a list of posts from the server and then pass the data to the component as props. The component can then be rendered on the server and reused on the client, allowing the list of posts to be updated interactively without requiring the entire page to be reloaded.
Here is an example of how to use the getInitialProps
method in a NEXT.js component:
import axios from 'axios'
function BlogList({ posts }) {
return (
<ul>
{posts.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
)
}
BlogList.getInitialProps = async () => {
const res = await axios.get('https://my-api.com/posts')
const data = res.data
return { posts: data }
}
export default BlogList
The getInitialProps
method is called when the BlogList
component is rendered on the server, and the data is fetched and passed to the component. The same data is reused when the component is later rendered on the client, allowing the component to be updated interactively without having to reload the entire page.
It is worth noting to note that the getInitialProps
function is only called on the server and the client, and it is not invoked during future client-side navigations. This implies that if you need to fetch data during client-side navigation, you'll have to choose an alternative technique. One approach is to utilize the getServerSideProps
function, which is identical to getInitialProps
but is called on every request, including client-side navigations.
Therefore, client-side rendering can be a valuable tool for increasing the efficiency of your NEXT.js project, and the getInitialProps
function is a critical component of that process. By retrieving data from the server and giving it to components as props, you may allow interactive changes without having to reload the entire page.
3. Use the next/head component
The next/head
component is a useful tool for optimizing the efficiency of your NEXT.js app by allowing you to modify the HTML document's <head>
element. This is particularly important for adding metadata and other SEO-related information. To use the next/head
component just import it as shown in the example below:
import Head from 'next/head'
function HomePage() {
return (
<div>
<Head>
<title>My App | Home</title>
<meta name="description" content="Welcome to my app" />
<link rel="canonical" href="https://my-app.com/" />
</Head>
<h1>Welcome to My App</h1>
</div>
)
}
export default HomePage
The NEXT.js Head component is used in the above example to add a title, a description, and a canonical link to the HTML document's head> element. These tags are vital for SEO and can assist enhance the performance and exposure of your app.
In addition to adding metadata and other information, the next/head component can also be used to add styles and scripts to your app. For example:
import Head from 'next/head'
function HomePage() {
return (
<div>
<Head>
<link rel="stylesheet" href="/static/css/main.css" />
<script src="/static/js/main.js" />
</Head>
<h1>Welcome to Next.js</h1>
</div>
)
}
export default HomePage
In this example, the Head component is used to add a stylesheet and a script to the <head>
element of the HTML document. This can be a useful way to include styles and scripts in your app without having to manually add them to each page or component.
Overall, the next/head component is a valuable tool for optimizing the performance of your NEXT.js app and can be used to manage the <head>
element of your HTML document conveniently and efficiently. Whether you're adding metadata, styles, or scripts, the next/head
component has you covered.
4. Optimize the loading of images
Image loading optimization is critical for ensuring a seamless user experience and boosting the speed of your NEXT.js project. Large or poorly optimized photos can drastically slow down page loading times, thus impacting user engagement and search engine rankings.
Using the built-in next/image
component to optimize image loading in your NEXT.js app is one option. This component optimizes photos automatically by converting them to the next-generation format (WebP
) and sending them at the correct size depending on the device's screen resolution. This can greatly reduce image file size and improve loading times.
Here is an example of how to use the next/image
component in a NEXT.js app:
- Import the next/image component at the top of your component file:
import Image from 'next/image'
- Use the
Image
component to render an image in your component's JSX:
<Image
src="/path/to/image-small.jpg"
srcSet={[
'/path/to/image-small.jpg 400w',
'/path/to/image-medium.jpg 800w',
'/path/to/image-large.jpg 1200w',
]}
sizes={`(max-width: 600px) 400px, 800px`}
alt="A description of the image"
width={1200}
height={600}
quality={75}
/>
Another technique for optimizing the loading of images is by implementing lazy loading with the IntersectionObserver API
. This API allows you only to load images when they are in the viewport, rather than loading all images on the page at once. This can significantly improve the initial loading time of your pages and save bandwidth for users.
In summary, to optimize the loading of images in your NEXT.js app, you can use the next/image
component for automatic optimization and the IntersectionObserver API
for lazy loading. By implementing these techniques, you can improve your app's performance and provide a better user experience.
5. Use a content delivery network (CDN)
Using a content delivery network (CDN) to distribute your assets and content over numerous servers in different locations is a powerful method to optimize the speed of your NEXT.js applications. This can assist minimize the burden on your servers while also improving the performance and dependability of your service for consumers worldwide.
There are many different CDN providers to choose from, each with its features and pricing plans. Some popular options include Cloudflare, Akamai, and Amazon CloudFront.
To use a CDN with your NEXT.js app, you normally configure it to serve assets and content from the CDN rather than your own servers. Depending on the CDN provider and the architecture of your app, this can be accomplished in a variety of ways.
One popular method is to use a reverse proxy, such as Cloudflare, to route traffic to your app through the CDN. This allows you to easily configure the CDN to cache assets and content, and to handle other performance-related tasks such as compression and minification.
Another method is to use a static asset hosting service, such as Amazon S3, to store and serve your app's assets and content directly from the CDN. This allows you to take advantage of the CDN's global network of servers, while also providing additional features such as automatic asset versioning and caching.
Here is an example of how to use a CDN with your NEXT.js app:
import Head from 'next/head'
function HomePage() {
return (
<div>
<Head>
<link rel="stylesheet" href="https://cdn.my-app.com/static/css/main.css" />
<script src="https://cdn.my-app.com/static/js/main.js" />
</Head>
<h1>Welcome to My App</h1>
</div>
)
}
export default HomePage
In this example, we are using a CDN to serve our stylesheet and script files by linking to them through the CDN's domain name "cdn.my-app.com" instead of serving them from our server. This way the files will be served to the user from the closest server to the user's location, which will increase the loading speed.
You can also instruct your CDN to cache your assets and content, allowing them to be served from the CDN's cache rather than your servers. This can minimize the burden on your servers while also increasing the speed of your app for consumers.
It is important to note that the process of integrating a CDN into your app may vary depending on the CDN provider and your app architecture. It is usually a good idea to consult the CDN provider's documentation and guidance for the best practices and specific steps to integrate them.
Overall, employing a CDN can be an effective approach to improve the performance of your NEXT.js app by reducing the burden on your servers, increasing speed and dependability, and decreasing latency for users all around the world. It is an efficient method for improving user experience and making your software scalable.
6. Enable compression and caching
Enabling compression and caching is an effective technique to improve the performance of your NEXT.js application by minimizing the amount of data transmitted to users and storing frequently used assets and content closer to the user. This can minimize the burden on your servers while also improving the performance and dependability of your service for consumers.
Compression is the process of reducing the size of data that is sent to users, such as text, images, and other assets. Compression can be enabled on your server or in your application and can be done using a variety of methods such as Gzip or Brotli. When enabled, compression can help reduce the amount of data that needs to be sent to users and can help improve the speed of your app.
Next.js provides gzip compression by default.
Caching refers to the process of storing frequently used assets and content closer to the user, such as on the user's browser or a CDN. Caching can be configured on your server, in your application, or on a CDN using a variety of techniques such as HTTP caching headers or service workers. When enabled, caching can assist reduce server load and enhance app performance.
Here is an example of how to enable caching in a NEXT.js app using the next-pwa
library:
const withPWA = require('next-pwa')
module.exports = withPWA({
pwa: {
runtimeCaching: [
{
urlPattern: /\.(png|jpg|jpeg|svg|gif)$/,
handler: 'CacheFirst'
}
]
}
})
In this example, the next-pwa
library is used to enable caching by creating a service worker that will cache image files.
It is important to note that the process of enabling compression and caching may vary depending on your server and app architecture. It's a good idea to consult the best practices and specific steps for your setup.
Overall, enabling compression and caching can be a powerful way to optimize the performance of your NEXT.js app by reducing the amount of data that needs to be sent to users and by storing frequently used assets and content closer to the user. This can help reduce the load on your servers and improve the speed and reliability of your app for users.
7. Monitor and analyze the performance
Monitoring and analyzing performance is a critical step in optimizing your NEXT.js app's performance. It enables you to identify and diagnose problems, track critical performance data, and assess the impact of app updates over time.
Google Analytics, New Relic, PageSpeed Insights and Google's Lighthouse are just a few of the tools and services you can use to monitor and analyze the performance of your apps. These tools enable you to track critical performance parameters like page load times, number of requests, and error rates, as well as provide thorough reports and insights that can assist you in identifying and diagnosing problems.
PageSpeed Insights, for example, may be used to do performance audits on your pages and provide detailed performance reports on the performance, accessibility, best practices, and SEO of your web pages. The report will highlight areas for improvement and provide concrete advise on how to address the issues.
Another method for monitoring performance is to log and debug the application; this offers you a better understanding of what's going on on the server and client sides, helping you to troubleshoot issues faster.
Browser dev tools can also be used to inspect network requests, analyze JS execution, and view displayed elements.
It is important to note that performance monitoring and analysis is a continuous process that requires constant monitoring and analysis to verify that your app is running well and to discover and diagnose issues as they develop.
Overall, performance monitoring and analysis are critical steps in enhancing the performance of your NEXT.js app. It allows you to detect and diagnose problems, track critical performance data, and assess the impact of app modifications over time. You can ensure that your app is running effectively and that any issues are swiftly detected and fixed by routinely monitoring and evaluating performance.
Furthermore, monitoring and evaluating performance can help you pinpoint areas for improvement, such as reducing load times, reducing the volume of requests, and increasing overall user experience. This can assist you in making data-driven decisions on how to enhance your app and guarantee it is performing optimally.
Setting up alerts and notifications to warn you of any performance issues or faults that arise is another option to monitor the performance of your app. This helps you to respond to issues quickly and resolve them before they affect your users.
When it comes to performance monitoring and analysis, it's essential to take a comprehensive approach by evaluating both the client-side and server-side aspects of your Next.js app. By doing so, you'll be able to pinpoint any problems that might arise and address them swiftly, regardless of whether they originate on the client or the server.
Conclusion
In summary, optimizing performance in Next.js is crucial for delivering a better experience to your users. The tips shared in this article will help boost your app's speed, stability & scalability. Experimentation and finding what works best for your app are key, and it's an ongoing process. Keep monitoring and fine-tuning your app for the best results.
Additional Resources
- Next.js documentation:
- Next.js GitHub repository:
- Next.js blog:
- Join the Next.js Discord:
- YouTube channel:
These resources will help you learn more about the different techniques and strategies that you can use to optimize the performance of your Next.js app, as well as provide you with examples and best practices to follow.