Create a Simple Web Server in Node

Davis Gitonga / January 14, 2022
3 min read •
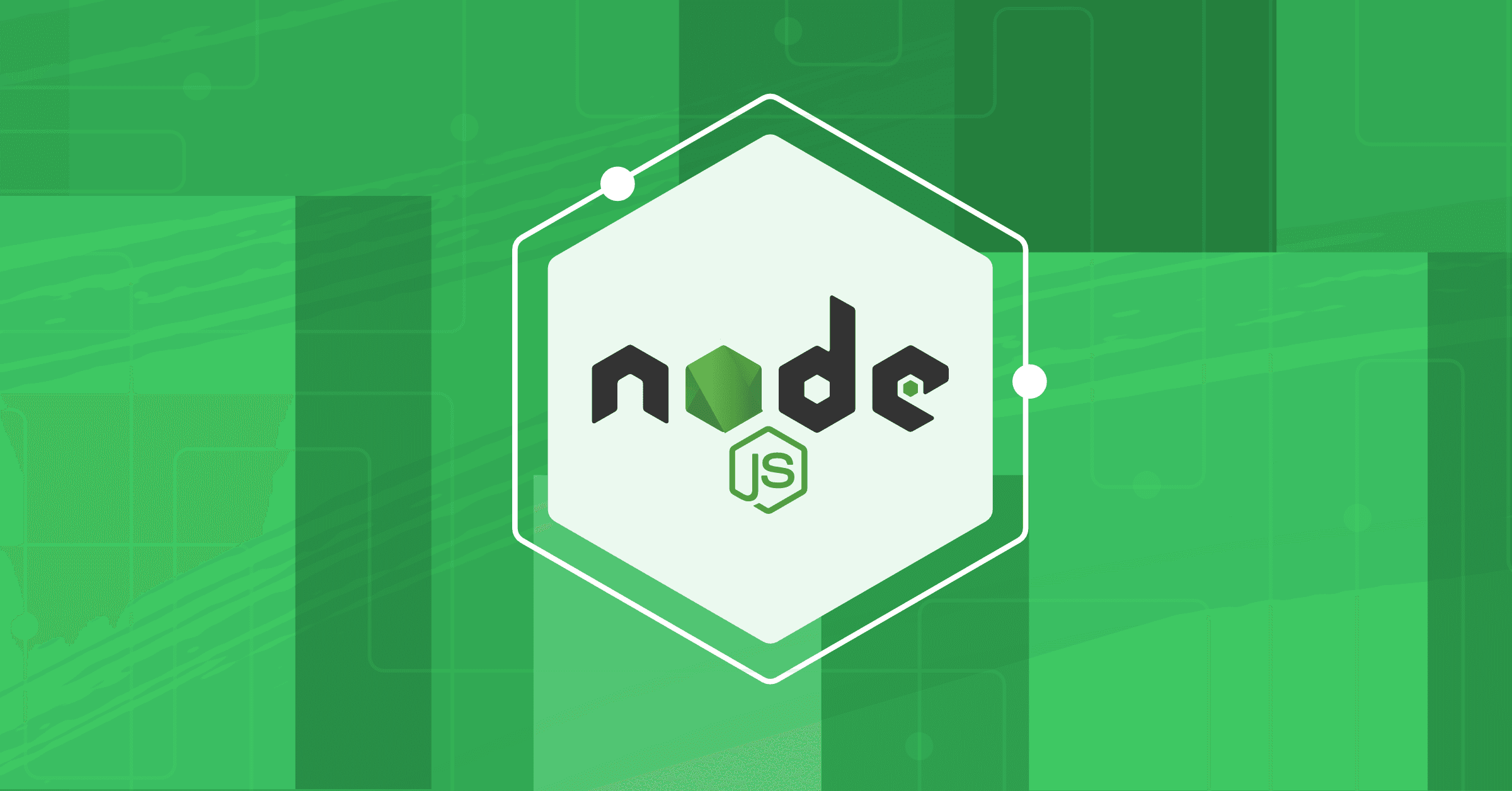
Introduction
In this article we are going to create a web server capable of accepting requests and sending back responses.
We will use the built-in http
node module which provides networking capabilities.
Prerequisites
You should be familiar with the basics of:
Additionally, you'll need to have Node installed on your local machine. Use
node -v
on the terminal to check your Node version.
Building the server
In order to build the server, we have to do two things. First we create the server and then second, we start the server so that we can actually listen to incoming requests.
Creating the server
Like i mentioned earlier, we will use the http
module which has a createServer
method on it.
const http = require('http');
const server = http.createServer((req, res) => {
res.end('Hello 👋 from the server!');
});
The createServer
method is an higher order function that accepts a callback function that gets called each time a new request hits our server.
The callback function gets access to two very important parameter objects named, request
and response
.
All we want to do now is to actually send back a response to the client. We do that with the response.end()
method.
This method signals to the server that all of the response headers and body have been sent; that server should consider this message complete. The method, response.end()
, MUST be called on each response.
Starting the server
Now we use our created server to call the listen
method which starts the HTTP server listening for connections from the client.
The listen
method accepts some parameters;
port
sub-address on a certain host.host
defaults tolocalhost
or127.0.0.1
which is the computer the program is running on.callback
function is optional and runs as soon as the server starts listening.
This function is asynchronous. When the server starts listening, the listening
event will be emitted. The last parameter callback
will be added as a listener for the listening
event.
Update the code to the following;
const http = require('http');
const port = 3000;
const server = http.createServer((req, res) => {
res.end('Hello from the server!');
});
server.listen(port, '127.0.0.1', () => {
console.log(`Server listening on port ${port}`);
});
Testing the server connection
All we have to do now is visit the host url 127.0.0.1
on the specificied port.
To do that, we first run the application with the node
command.
node server.js
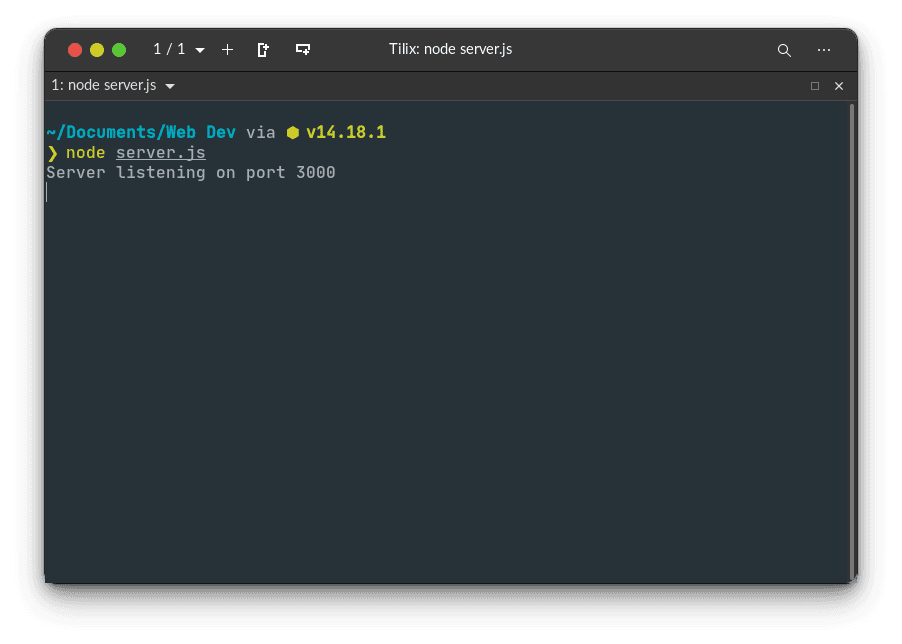
We then navigate to our broswer and send a request to the server.
The image below shows the response from the server.
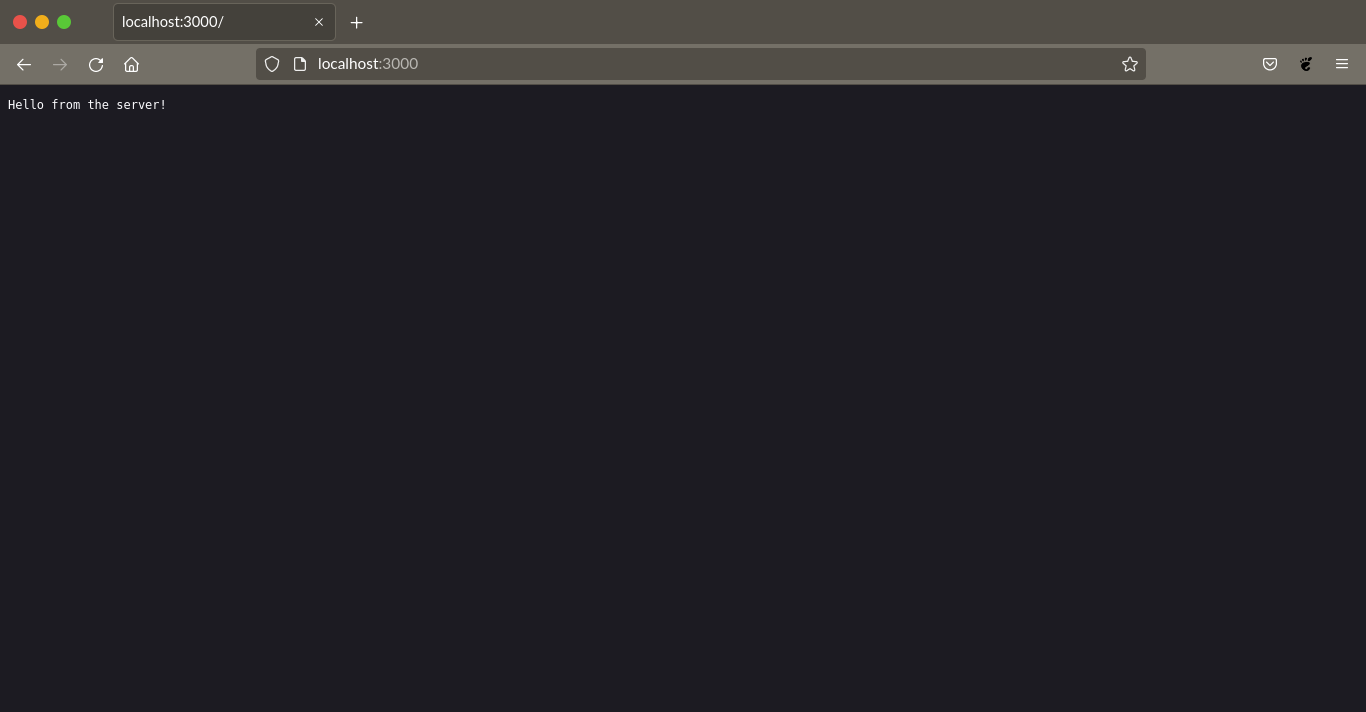
Conclusion
That is one of the easiest ways to build a server in node.
Thanks for reading! If this tutorial was helpful, try it out yourself and share your feedback in the comments section below.
Happy Coding!🤓